- Branch and Bound Tutorial
- Backtracking Vs Branch-N-Bound
- 0/1 Knapsack
- 8 Puzzle Problem
- Job Assignment Problem
- N-Queen Problem
- Travelling Salesman Problem

Job Assignment Problem using Branch And Bound
Let there be N workers and N jobs. Any worker can be assigned to perform any job, incurring some cost that may vary depending on the work-job assignment. It is required to perform all jobs by assigning exactly one worker to each job and exactly one job to each agent in such a way that the total cost of the assignment is minimized.
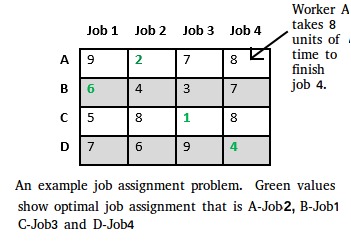
Let us explore all approaches for this problem.
Solution 1: Brute Force
We generate n! possible job assignments and for each such assignment, we compute its total cost and return the less expensive assignment. Since the solution is a permutation of the n jobs, its complexity is O(n!).
Solution 2: Hungarian Algorithm
The optimal assignment can be found using the Hungarian algorithm. The Hungarian algorithm has worst case run-time complexity of O(n^3).
Solution 3: DFS/BFS on state space tree
A state space tree is a N-ary tree with property that any path from root to leaf node holds one of many solutions to given problem. We can perform depth-first search on state space tree and but successive moves can take us away from the goal rather than bringing closer. The search of state space tree follows leftmost path from the root regardless of initial state. An answer node may never be found in this approach. We can also perform a Breadth-first search on state space tree. But no matter what the initial state is, the algorithm attempts the same sequence of moves like DFS.

Solution 4: Finding Optimal Solution using Branch and Bound
The selection rule for the next node in BFS and DFS is “blind”. i.e. the selection rule does not give any preference to a node that has a very good chance of getting the search to an answer node quickly. The search for an optimal solution can often be speeded by using an “intelligent” ranking function, also called an approximate cost function to avoid searching in sub-trees that do not contain an optimal solution. It is similar to BFS-like search but with one major optimization. Instead of following FIFO order, we choose a live node with least cost. We may not get optimal solution by following node with least promising cost, but it will provide very good chance of getting the search to an answer node quickly.
There are two approaches to calculate the cost function:
- For each worker, we choose job with minimum cost from list of unassigned jobs (take minimum entry from each row).
- For each job, we choose a worker with lowest cost for that job from list of unassigned workers (take minimum entry from each column).
In this article, the first approach is followed.
Let’s take below example and try to calculate promising cost when Job 2 is assigned to worker A.
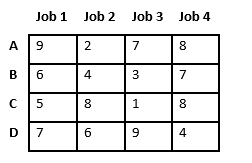
Since Job 2 is assigned to worker A (marked in green), cost becomes 2 and Job 2 and worker A becomes unavailable (marked in red).
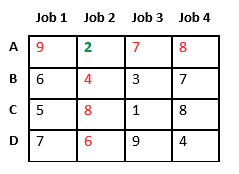
Now we assign job 3 to worker B as it has minimum cost from list of unassigned jobs. Cost becomes 2 + 3 = 5 and Job 3 and worker B also becomes unavailable.
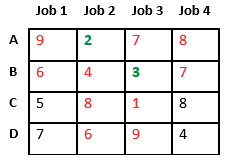
Finally, job 1 gets assigned to worker C as it has minimum cost among unassigned jobs and job 4 gets assigned to worker D as it is only Job left. Total cost becomes 2 + 3 + 5 + 4 = 14.
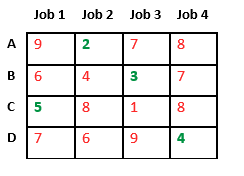
Below diagram shows complete search space diagram showing optimal solution path in green.
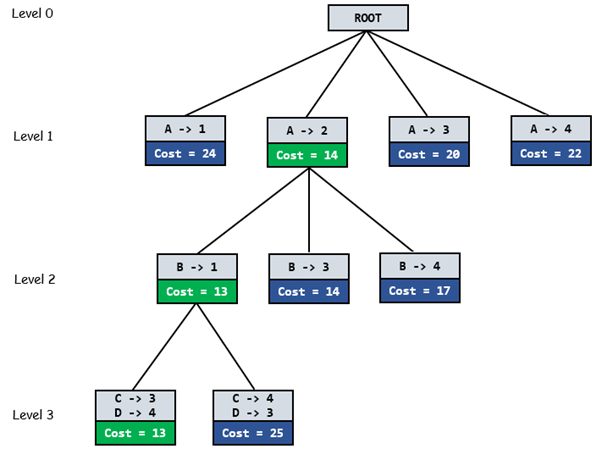
Complete Algorithm:
Below is the implementation of the above approach:
Time Complexity: O(M*N). This is because the algorithm uses a double for loop to iterate through the M x N matrix. Auxiliary Space: O(M+N). This is because it uses two arrays of size M and N to track the applicants and jobs.
Similar Reads
- Branch and Bound Algorithm The Branch and Bound Algorithm is a method used in combinatorial optimization problems to systematically search for the best solution. It works by dividing the problem into smaller subproblems, or branches, and then eliminating certain branches based on bounds on the optimal solution. This process c 1 min read
- Introduction to Branch and Bound - Data Structures and Algorithms Tutorial Branch and bound algorithms are used to find the optimal solution for combinatory, discrete, and general mathematical optimization problems. A branch and bound algorithm provide an optimal solution to an NP-Hard problem by exploring the entire search space. Through the exploration of the entire sear 13 min read
- 0/1 Knapsack using Branch and Bound Given two arrays v[] and w[] that represent values and weights associated with n items respectively. Find out the maximum value subset(Maximum Profit) of v[] such that the sum of the weights of this subset is smaller than or equal to Knapsack capacity W. Note: The constraint here is we can either pu 15+ min read
- Implementation of 0/1 Knapsack using Branch and Bound Given two arrays v[] and w[] that represent values and weights associated with n items respectively. Find out the maximum value subset(Maximum Profit) of v[] such that the sum of the weights of this subset is smaller than or equal to Knapsack capacity Cap(W). Note: The constraint here is we can eith 15+ min read
- 8 puzzle Problem Given a 3×3 board with 8 tiles (each numbered from 1 to 8) and one empty space, the objective is to place the numbers to match the final configuration using the empty space. We can slide four adjacent tiles (left, right, above, and below) into the empty space. 1. 8 puzzle Problem using DFS (Brute-Fo 15+ min read
- Job Assignment Problem using Branch And Bound Let there be N workers and N jobs. Any worker can be assigned to perform any job, incurring some cost that may vary depending on the work-job assignment. It is required to perform all jobs by assigning exactly one worker to each job and exactly one job to each agent in such a way that the total cost 15+ min read
- N Queen Problem using Branch And Bound The N queens puzzle is the problem of placing N chess queens on an N×N chessboard so that no two queens threaten each other. Thus, a solution requires that no two queens share the same row, column, or diagonal. The backtracking Algorithm for N-Queen is already discussed here. In a backtracking solut 15+ min read
- Traveling Salesman Problem using Branch And Bound Given a set of cities and distance between every pair of cities, the problem is to find the shortest possible tour that visits every city exactly once and returns to the starting point. For example, consider the graph shown in figure on right side. A TSP tour in the graph is 0-1-3-2-0. The cost of t 15+ min read
- Branch and Bound
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

IMAGES
VIDEO